WP_Query is a PHP class in WordPress which loads content from the database. Developers rely on WP_Query to build custom lists of content that display on the site’s front-end.
To understand how WP_Query works, let’s look at an example. Here is a page that displays three “promoted” tours:
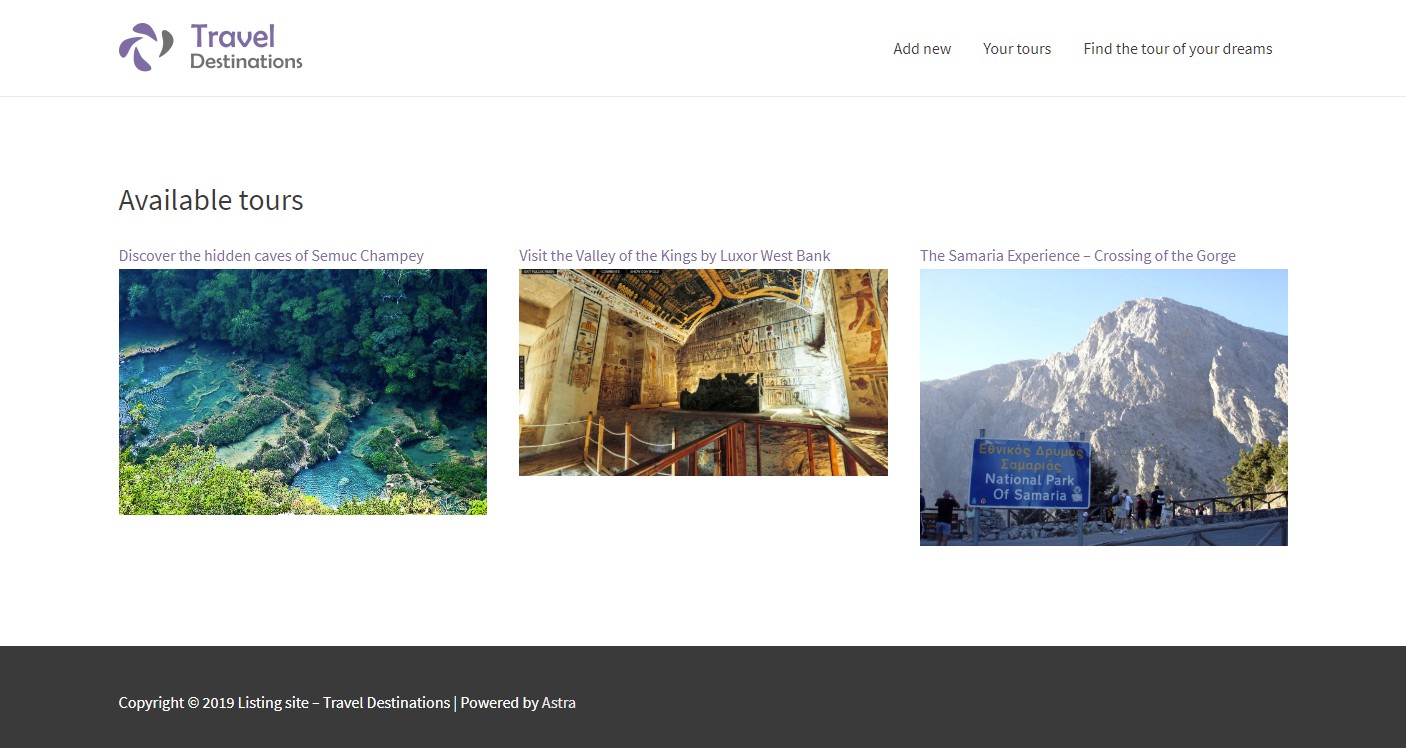
You need to use WP_Query to load these tour posts from the database. We will show how to create this list in two ways:
- Using WP_Query via PHP code (requires programming)
- Using WP_Query using the Toolset GUI (no programming needed)
Using WP_Query with PHP code
The code below is the query part. Here, WP_Query loads three random “tours” from the database which match the criteria.
First, the code constructs an array of arguments ($args), which includes all the details of the query that we need to run. Then, we call the WP_Query class and ask it to return the items (tours) that match the arguments.
function display_promoted_tours(){ // Create an array of arguments, which will tell WP_Query what information to load from the database $args = array( 'post_type' => 'tours', 'post_status' => 'publish', 'limit' => 3, 'orderby' => 'rand', 'tax_query' => array( 'relation' => 'AND', array( 'taxonomy' => 'tour-status', 'field' => 'slug', 'terms' => array( 'promoted' ), 'operator' => 'IN' ) ), 'meta_query' = array( 'relation' => 'AND', array( 'key' => 'wpcf-valid-until', 'value' => strtotime( 'today 00:00' ), 'type' => 'NUMERIC', 'compare' = '>' ) ) ); // Call WP_Query and load the content from the database $my_query = new WP_Query( $args ); // Now display the “tours” if ( $my_query->have_posts() ) : while ( $my_query->have_posts() ) : $my_query->the_post(); echo " <div class='card'> "; if ( has_post_thumbnail() ) : echo "<a href='" . get_the_permalink() . "' title='" . the_title(null,null,false) . "'>" . get_the_post_thumbnail() . "</a>"; endif; echo the_title( ' <h3>', '</h3> ', false); echo "<button class='button-style'><a href='" . get_the_permalink() . "'>Learn more</button>"; echo "</div> "; endwhile; wp_reset_postdata(); endif; }
Now, you have the code that loads the content from the database and displays it on the front-end. The last step is to add this code to one of your theme’s templates, so that it will run on the page where you want it to appear.
To do this, you will need to follow these additional steps:
- Create a child theme, so that you don’t directly edit the theme’s files (causing future update problems)
- Copy the theme’s page.php template and rename it
- Edit the new template file and call display_promoted_tours from it
- From the WordPress admin, edit the page which should show this list of tours and set it to use the new template
This process works fine, but as you can see it requires a fair amount of coding and debugging. If you’re interested in the PHP-free version of this process, which takes minutes instead of days, continue reading.
Create a query with Toolset GUI
Let’s use the same travel website example as above and create a query using Toolset instead of PHP coding.
Below is the same example as above but this time without using coding – just Toolset.
- Insert the Views editor block where you want the list to appear
- Choose what content you want to load
- Design how the list will look
In this example, the View displays the query results as a grid. Your Views can display results in any way, even as markers on Maps.
Adding pagination to queries
When you want to create a long list of content you can break it down by adding pagination.
To show results in pages, instead of one long list, you will need to:
Creating custom searches
Besides displaying the results as a list you can also use queries to build custom searches. Custom search adds front-end controls that allow users to filter the results and find the exact content they are looking for.
Displaying query results on a map
If your posts have a custom Address field, you can display them on a map.
Limiting and filtering queries
The queries that you build don’t have to load from the database all the items of the type that you’ve selected. You can limit and filter queries to return the exact results that you want to get.
For example, you can create queries that will:
- Return the first several results
- Filter the results according to the values of custom fields and taxonomy
- Filter the results according to post relationship
- Filter the results according to inputs on the front-end (create a custom search)